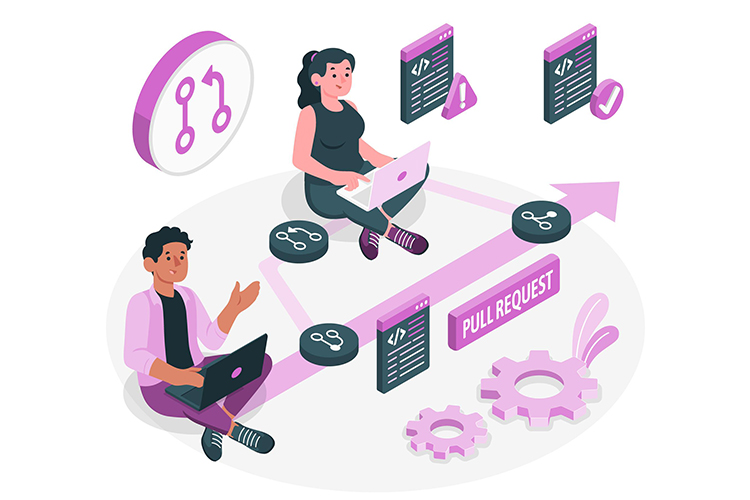
Introduction
Nested routing in React.js allows you to create complex and dynamic user interfaces by organizing your application into smaller, manageable components. Advanced concepts in mastering nested routing involve deeper understanding of React Router, component composition, and state management. Below are some advanced concepts to consider when working with nested routing in React:
Dynamic Route Matching
Use dynamic route parameters to create flexible and reusable components. For example, if you have a user profile page, the route might look like /users/:userId
. You can then extract the userId
from the URL parameters and use it to fetch user-specific data.
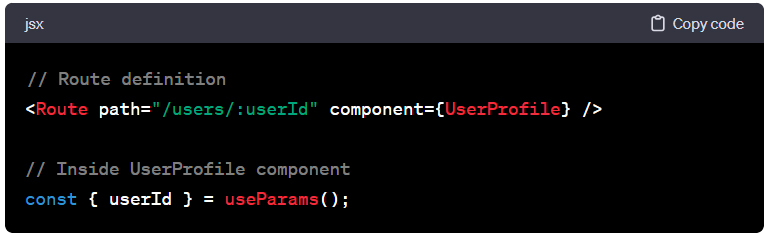
Nested Route Components:
Break down your UI into smaller components and nest them within the corresponding route components. This promotes component reusability and keeps your codebase organized.
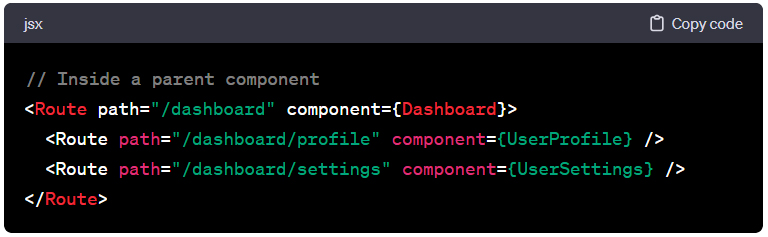
Render Prop Component:
Instead of using the component
prop, use the render
prop with a function. This allows you to pass additional props to the rendered component and perform logic before rendering.

Route Guards and Authentication
Implement route guards to control access to certain routes based on user authentication or other conditions. You can use higher-order components or render props to conditionally render components.
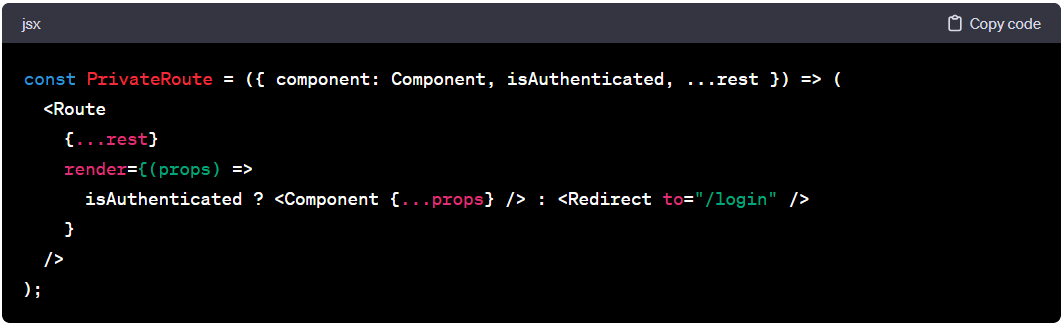
Programmatic Navigation
Use the useHistory
hook or withRouter
HOC to programmatically navigate between routes. This is useful for redirecting users after certain actions or implementing dynamic navigation logic.
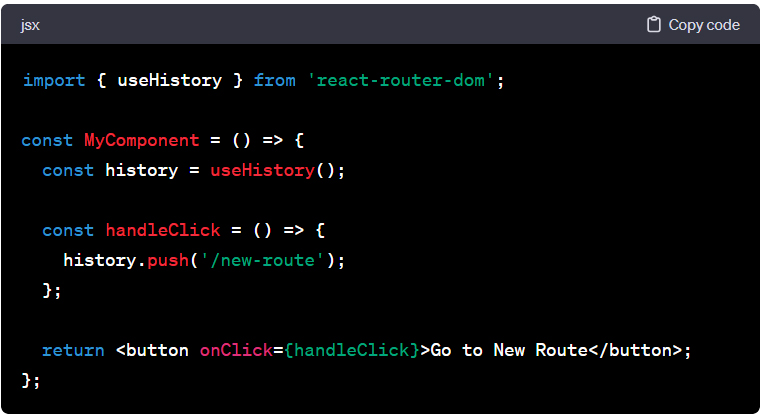
State Management Integration
Integrate your nested routing with state management libraries like Redux or Context API. This ensures that state is shared and synchronized across components, even those at different levels of nesting.
Error Handling and Fallback Routes
Implement error boundaries for your route components to gracefully handle errors. Additionally, use fallback routes (<Route path="*" />
) to display a custom error page or redirect users when they access an undefined route.

Lazy Loading and Code Splitting
Improve performance by employing code splitting and lazy loading for your route components. This allows you to load only the necessary code when a specific route is accessed, reducing initial page load times.
Animated Transitions
Add animated transitions between different route components for a smoother user experience. Libraries like react-transition-group
can help you achieve this.
Testing Nested Routes
Write comprehensive unit tests and integration tests for your nested routes. Consider using testing libraries like Jest and React Testing Library to ensure the reliability of your routing logic.
Infermation
Services
Contact Us
- +91 7205154482
- info@alysiancreative.com
- #H1/1, Ground floor, Arya Village, Panchasakha nagar, Near Beleswar Shiv Temple, Bhubaneswar, Odisha - 751019
Subscribe Newsletter
Copyright © 2024 Proudly powered by Alysian Creative All rights reserved.